Doug Johnson
New Member
(Progress Version 9.1E/Windows XP)
I have an initial frame containing the following fields:
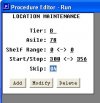
After the user enters values into the fields, they can click "Add". Upon clicking "Add", the following frame is displayed:
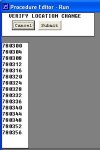
The user then decides if they want to "Cancel" the addition or "Submit" the addition.
Problems:
1.) When the user enters the values in frame1, they have to hit (F2) "BEFORE" they can hit the "ADD" button. This is because in my code I am using an UPDATE statement to update all of the fields. Until the user hits (F2) the button CHOOSE statements aren't enabled. Is there another way to update fields that allows the buttons to remain active without a (F2)? (SEE CODE BELOW)
2.) When the user does hit the "ADD" button in frame1, and then hit's the "CANCEL" or "SUBMIT" buttons on frame2, they are returned to frame1, but the previous values are still in the frame1 fields AND even if they are changed, the previous values are being passed to frame2. (SEE CODE BELOW)
Hopefully you'll be able to run this code yourself.
I have an initial frame containing the following fields:
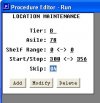
After the user enters values into the fields, they can click "Add". Upon clicking "Add", the following frame is displayed:
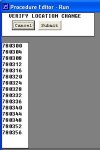
The user then decides if they want to "Cancel" the addition or "Submit" the addition.
Problems:
1.) When the user enters the values in frame1, they have to hit (F2) "BEFORE" they can hit the "ADD" button. This is because in my code I am using an UPDATE statement to update all of the fields. Until the user hits (F2) the button CHOOSE statements aren't enabled. Is there another way to update fields that allows the buttons to remain active without a (F2)? (SEE CODE BELOW)
2.) When the user does hit the "ADD" button in frame1, and then hit's the "CANCEL" or "SUBMIT" buttons on frame2, they are returned to frame1, but the previous values are still in the frame1 fields AND even if they are changed, the previous values are being passed to frame2. (SEE CODE BELOW)
Hopefully you'll be able to run this code yourself.
Code:
DEFINE TEMP-TABLE whstore NO-UNDO
FIELD zone AS CHAR
FIELD whse-loc AS CHAR
FIELD skid-color AS CHAR.
DEFINE VARIABLE tier AS CHARACTER FORMAT "X(2)".
DEFINE VARIABLE finallocation AS CHARACTER FORMAT "X(8)".
DEFINE VARIABLE asile AS CHARACTER FORMAT "X(2)".
DEFINE VARIABLE shelffrom AS INTEGER FORMAT "9".
DEFINE VARIABLE shelfto AS INTEGER FORMAT "9".
DEFINE VARIABLE levelloop AS INTEGER FORMAT "9".
DEFINE VARIABLE locationloop AS INTEGER FORMAT "999".
DEFINE VARIABLE startpos AS INTEGER FORMAT "999".
DEFINE VARIABLE stoppos AS INTEGER FORMAT "999".
DEFINE VARIABLE skippos AS INTEGER FORMAT "99".
DEFINE BUTTON newlocationbutton
LABEL "Add"
FONT 2
SIZE 5 BY 1.5.
DEFINE BUTTON cancel
LABEL "Cancel"
FONT 2
SIZE 7 BY 1.5.
DEFINE BUTTON submit
LABEL "Submit"
FONT 2
SIZE 7 BY 1.5.
DEFINE FRAME frame1
" LOCATION MAINTENANCE " AT 2 SKIP(.25)
SKIP(1)
"Tier:" AT 9 tier AT 15 SKIP(.5)
"Asile:" AT 8 asile AT 15 SKIP(.5)
"Shelf Range:" AT 2
shelffrom AT 15
"<->" AT 17
shelfto AT 21 SKIP(.5)
"Start/Stop:" AT 3
startpos AT 15
"<->" AT 19
stoppos AT 23 SKIP(.5)
"Skip:" AT 9 skippos AT 15 SKIP(1)
SPACE(1) newlocationbutton AT 3 SKIP(1)
WITH NO-LABELS.
DEFINE FRAME frame2
" VERIFY LOCATION CHANGE " AT 2 SKIP(.25)
SPACE(1) cancel AT 4
SPACE(1) submit SKIP(1)
WITH NO-LABELS.
/*###### PROCEDURES #########*/
/*##### ADD LOCATION #####*/
PROCEDURE addlocation:
ON CHOOSE OF cancel IN FRAME frame2 DO:
APPLY "U1" TO THIS-PROCEDURE.
END.
ON CHOOSE OF submit IN FRAME frame2 DO:
APPLY "U1" TO THIS-PROCEDURE.
END.
ENABLE ALL WITH FRAME frame2.
levelloop = 0.
DO WHILE levelloop LE shelfto:
locationloop = startpos.
DO WHILE locationloop LE stoppos:
finallocation = STRING(asile) + STRING(levelloop) + STRING(locationloop).
DISPLAY finallocation NO-LABELS WITH DOWN FRAME a.
DOWN WITH FRAME a.
locationloop = locationloop + skippos.
END.
levelloop = levelloop + 1.
END.
ENABLE ALL WITH FRAME frame2.
WAIT-FOR "U1" OF THIS-PROCEDURE.
HIDE FRAME frame2.
VIEW FRAME frame1.
END PROCEDURE.
/*####### MAIN PROGRAM ######*/
REPEAT:
UPDATE tier asile shelffrom shelfto startpos stoppos skippos newlocationbutton WITH FRAME frame1.
ON CHOOSE OF newlocationbutton IN FRAME frame1 DO:
HIDE FRAME frame1.
RUN addlocation.
END.
ENABLE ALL WITH FRAME frame1.
WAIT-FOR WINDOW-CLOSE OF CURRENT-WINDOW.
END.